Email Regular Expression
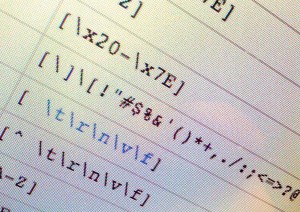
You might consider this a cynical view, but you should probably not try to implement a very strict email validation pattern with regular expressions. The fully compliant RFC-822 email regex is nothing to be trifled with; in fact, it is a behemoth.
As such, I recommend using only a simple regular expression for this task. And iIf you need to validate users email addresses, consider sending them a verification to confirm that what they have given you is correct, but if you really want to be strict about things – consider a pre-built validation package.
The pattern:
^\S+@\S+\.\S+$ #just make sure that it "pretty much" looks like an email address |
Validation in Java:
In Java, to be more comprehensive and accurate, you could use the following technique provided by the “javax.mail” specification:
public static boolean isValidEmailAddress(String email) { boolean result = true; try { InternetAddress emailAddr = new InternetAddress(email); emailAddr.validate(); } catch (AddressException ex) { result = false; } return result; }
Validation in PHP
PHP also offers a built-in validation mechanism via the filter_var
function.
<?php $email = "someone@exa mple.com"; if(!filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "E-mail is not valid"; } else { echo "E-mail is valid"; } ?>
[…] 10. Email Regexp | Validate Email Java | Regular Expression for … […]
[…] 10. Email Regexp | Validate Email Java | Regular Expression for … […]
[…] 10. Regexp Email | Check Java Email | Regular Expression for … […]
[…] 10. Email Regexp | Validate Email Java | Regular Expression for … […]